TypeScript Type Changes in React 18
2022. 4. 28.
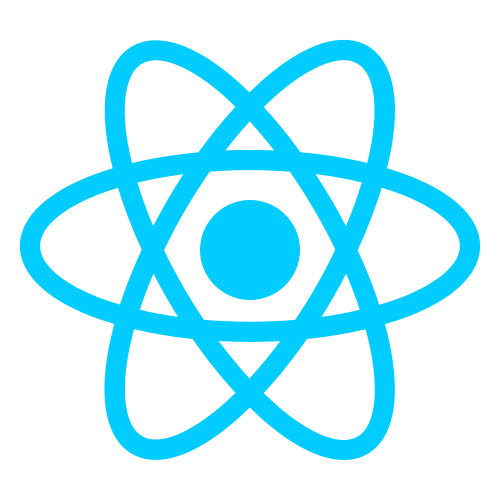
React 18 was released recently. You might have already updated your project or are planning to update soon.
If your project uses React with TypeScript, here are a few breaking changes in type definitions that you'll need to address:
- Removal of implicit
children
declaration from theReact.FC
type - Removal of
{}
from theReactFragment
type this.context
is now theunknown
type
You'll likely search for these anyway. It's good to be aware of them beforehand :)
Removal of Implicit children
Declaration in React.FC
Type
This might surprise some people. If you started with JavaScript and React and later adopted TypeScript, one of the first things you likely searched for was how to declare children
as a prop. You probably solved it by using React.FC
. However, all children
-related declarations are now removed from React.FC
.
So, as soon as you update React, existing code that used children
will likely show errors.
const Example: React.FC = ({ children }) => <div>{children}</div>;
// "TS2339: Property 'children' does not exist on type '{}'."
It might seem inconvenient, but the implicit nature of children
posed a significant problem from a type perspective: it couldn't clearly express whether child nodes were required or not. The drawback was that types couldn't catch cases where child nodes were omitted from components requiring them, or vice versa.
Therefore, the intention is to explicitly declare children
according to the component's needs, instead of relying on the implicit declaration. I strongly agree with this.
Like this:
type Props = {
children: React.ReactNode;
};
const Example: React.FC<Props> = ({ children }) => <div>{children}</div>;
This component's type definition now explicitly states that child components are required.
If child nodes are optional, you can declare them as optional, right?
type Props = {
children?: React.ReactNode;
};
Previously, children
was likely optional within the old React.FC
as well.
If you don't want to declare it like this every time but still want a somewhat standard approach, you can use PropsWithChildren
.
type Props = {
title?: string;
};
const Example: React.FC<React.PropsWithChildren<Props>> = ({ children }) => <div>{children}</div>;
However, the code feels a bit more complex. Nested generics tend to increase complexity. I prefer declaring children
explicitly as needed, aligning with the intent of this change.
Removal of {}
from ReactFragment
Type
This is an additional change resulting from the children
modification, but you don't necessarily need to know the details. The {}
(object) type has been removed from the ReactFragment
type. Creating child nodes with plain objects hasn't been supported since React 14, but it was kept in the types because of the implicit children
. It has now been removed along with the children
change.
this.context
is Now unknown
Type
Previously, it was of type any
, which was ambiguous and not type-friendly. Now, it's unknown
. You must explicitly cast it to a specific type before using it. While there might be ways to revert to any
, you probably don't need to know them unless you have a specific edge case.
with kakaopay
Recommend Post
This work is licensed under a Creative Commons Attribution-NonCommercial-NoDerivatives 4.0 International License.